Table Builder
Getting started
Overview
Filament's Table Builder package allows you to add an interactive datatable to any Livewire component. It's also used within other Filament packages, such as the Panel Builder for displaying resources and relation managers, as well as for the table widget. Learning the features of the Table Builder will be incredibly time-saving when both building your own custom Livewire tables and using Filament's other packages.
This guide will walk you through the basics of building tables with Filament's table package. If you're planning to add a new table to your own Livewire component, you should do that first and then come back. If you're adding a table to an app resource, or another Filament package, you're ready to go!
Defining table columns
The basis of any table is rows and columns. Filament uses Eloquent to get the data for rows in the table, and you are responsible for defining the columns that are used in that row.
Filament includes many column types prebuilt for you, and you can view a full list here. You can even create your own custom column types to display data in whatever way you need.
Columns are stored in an array, as objects within the $table->columns()
method:
use Filament\Tables\Columns\IconColumn;use Filament\Tables\Columns\TextColumn;use Filament\Tables\Table; public function table(Table $table): Table{ return $table ->columns([ TextColumn::make('title'), TextColumn::make('slug'), IconColumn::make('is_featured') ->boolean(), ]);}
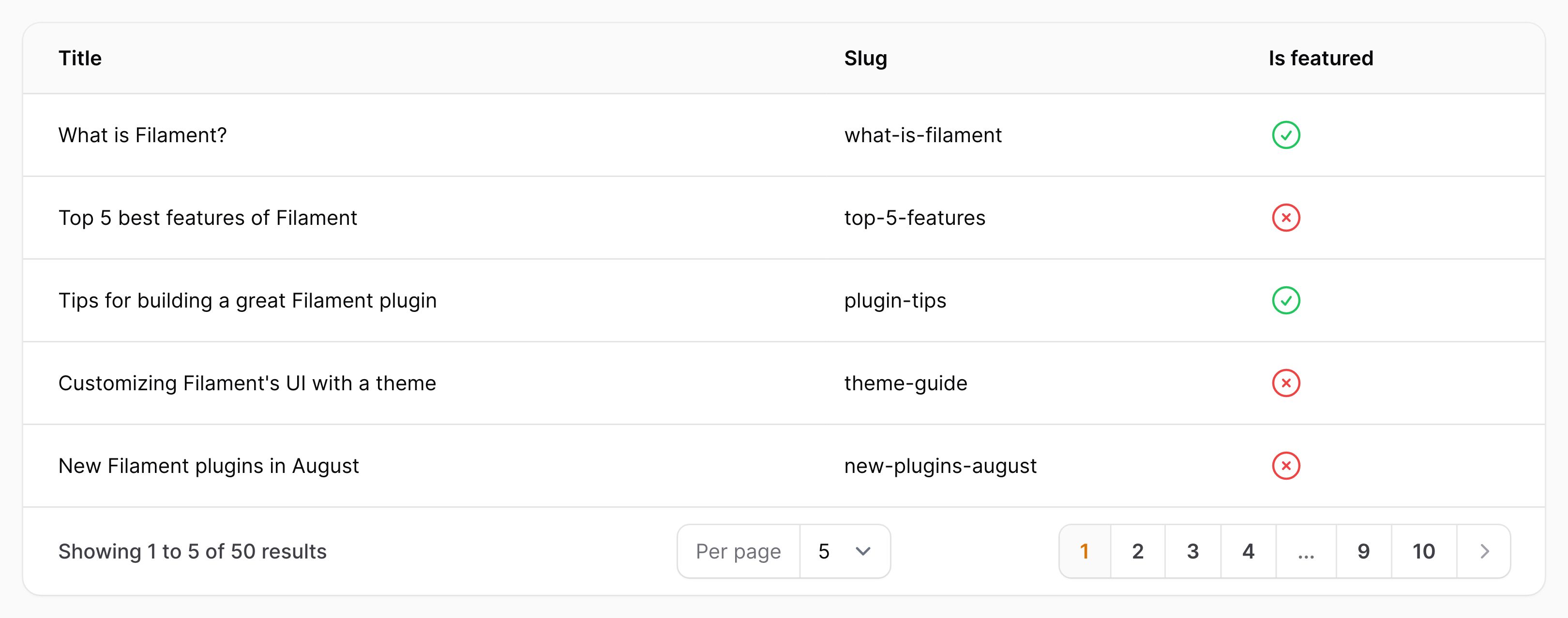
In this example, there are 3 columns in the table. The first two display text - the title and slug of each row in the table. The third column displays an icon, either a green check or a red cross depending on if the row is featured or not.
Making columns sortable and searchable
You can easily modify columns by chaining methods onto them. For example, you can make a column searchable using the searchable()
method. Now, there will be a search field in the table, and you will be able to filter rows by the value of that column:
use Filament\Tables\Columns\TextColumn; TextColumn::make('title') ->searchable()

You can make multiple columns searchable, and Filament will be able to search for matches within any of them, all at once.
You can also make a column sortable using the sortable()
method. This will add a sort button to the column header, and clicking it will sort the table by that column:
use Filament\Tables\Columns\TextColumn; TextColumn::make('title') ->sortable()

Accessing related data from columns
You can also display data in a column that belongs to a relationship. For example, if you have a Post
model that belongs to a User
model (the author of the post), you can display the user's name in the table:
use Filament\Tables\Columns\TextColumn; TextColumn::make('author.name')
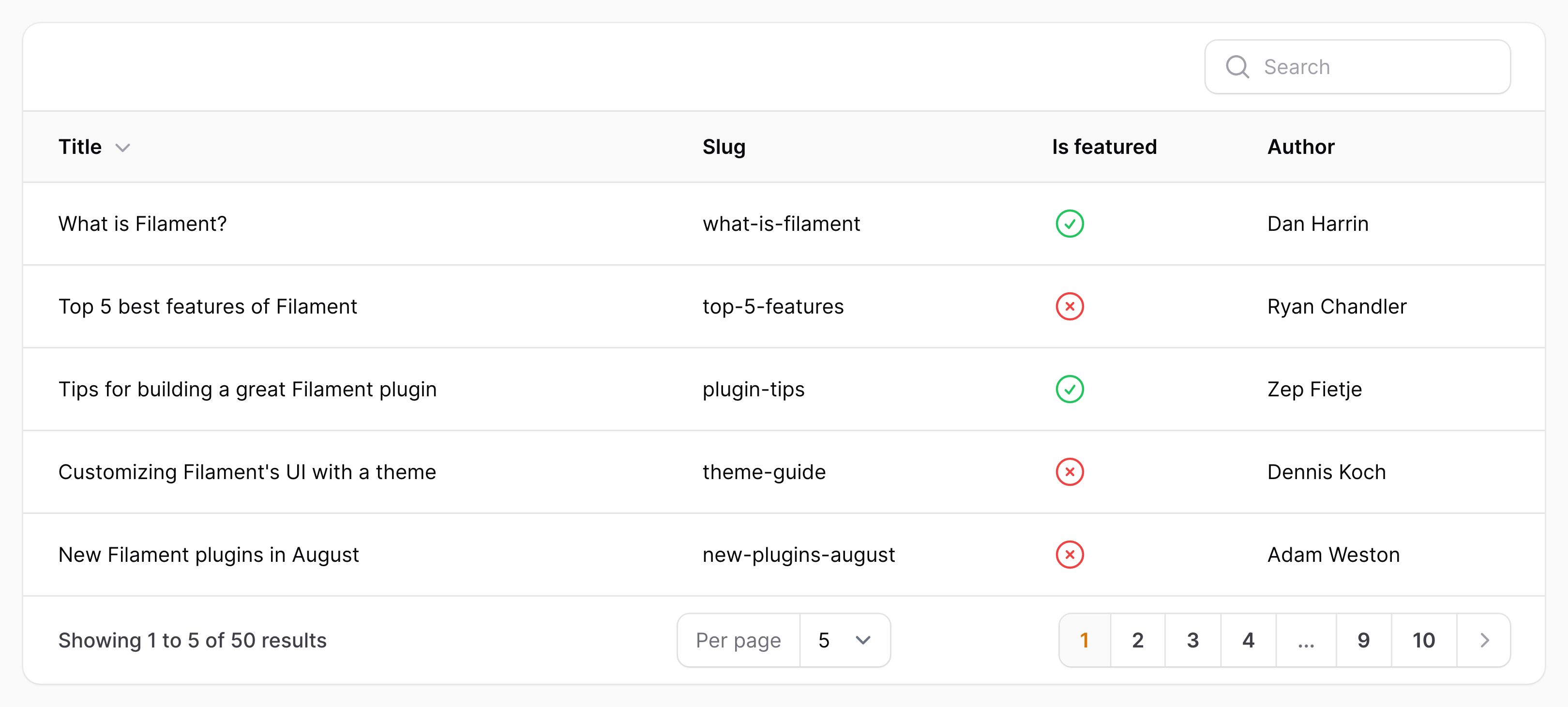
In this case, Filament will search for an author
relationship on the Post
model, and then display the name
attribute of that relationship. We call this "dot notation" - you can use it to display any attribute of any relationship, even nested distant relationships. Filament uses this dot notation to eager-load the results of that relationship for you.
Defining table filters
As well as making columns searchable()
, you can allow the users to filter rows in the table in other ways. We call these components "filters", and they are defined in the $table->filters()
method:
use Filament\Tables\Filters\Filter;use Filament\Tables\Filters\SelectFilter;use Filament\Tables\Table;use Illuminate\Database\Eloquent\Builder; public function table(Table $table): Table{ return $table ->columns([ // ... ]) ->filters([ Filter::make('is_featured') ->query(fn (Builder $query) => $query->where('is_featured', true)), SelectFilter::make('status') ->options([ 'draft' => 'Draft', 'reviewing' => 'Reviewing', 'published' => 'Published', ]), ]);}

In this example, we have defined 2 table filters. On the table, there is now a "filter" icon button in the top corner. Clicking it will open a dropdown with the 2 filters we have defined.
The first filter is rendered as a checkbox. When it's checked, only featured rows in the table will be displayed. When it's unchecked, all rows will be displayed.
The second filter is rendered as a select dropdown. When a user selects an option, only rows with that status will be displayed. When no option is selected, all rows will be displayed.
It's possible to define as many filters as you need, and use any component from the Form Builder package to create a UI. For example, you could create a custom date range filter.
Defining table actions
Filament's tables can use Actions. They are buttons that can be added to the end of any table row, or even in the header of a table. For instance, you may want an action to "create" a new record in the header, and then "edit" and "delete" actions on each row. Bulk actions can be used to execute code when records in the table are selected.
use App\Models\Post;use Filament\Tables\Actions\Action;use Filament\Tables\Actions\BulkActionGroup;use Filament\Tables\Actions\DeleteBulkAction; public function table(Table $table): Table{ return $table ->columns([ // ... ]) ->actions([ Action::make('feature') ->action(function (Post $record) { $record->is_featured = true; $record->save(); }) ->hidden(fn (Post $record): bool => $record->is_featured), Action::make('unfeature') ->action(function (Post $record) { $record->is_featured = false; $record->save(); }) ->visible(fn (Post $record): bool => $record->is_featured), ]) ->bulkActions([ BulkActionGroup::make([ DeleteBulkAction::make(), ]), ]);}

In this example, we define 2 actions for table rows. The first action is a "feature" action. When clicked, it will set the is_featured
attribute on the record to true
- which is written within the action()
method. Using the hidden()
method, the action will be hidden if the record is already featured. The second action is an "unfeature" action. When clicked, it will set the is_featured
attribute on the record to false
. Using the visible()
method, the action will be hidden if the record is not featured.
We also define a bulk action. When bulk actions are defined, each row in the table will have a checkbox. This bulk action is built-in to Filament, and it will delete all selected records. However, you can write your own custom bulk actions easily too.
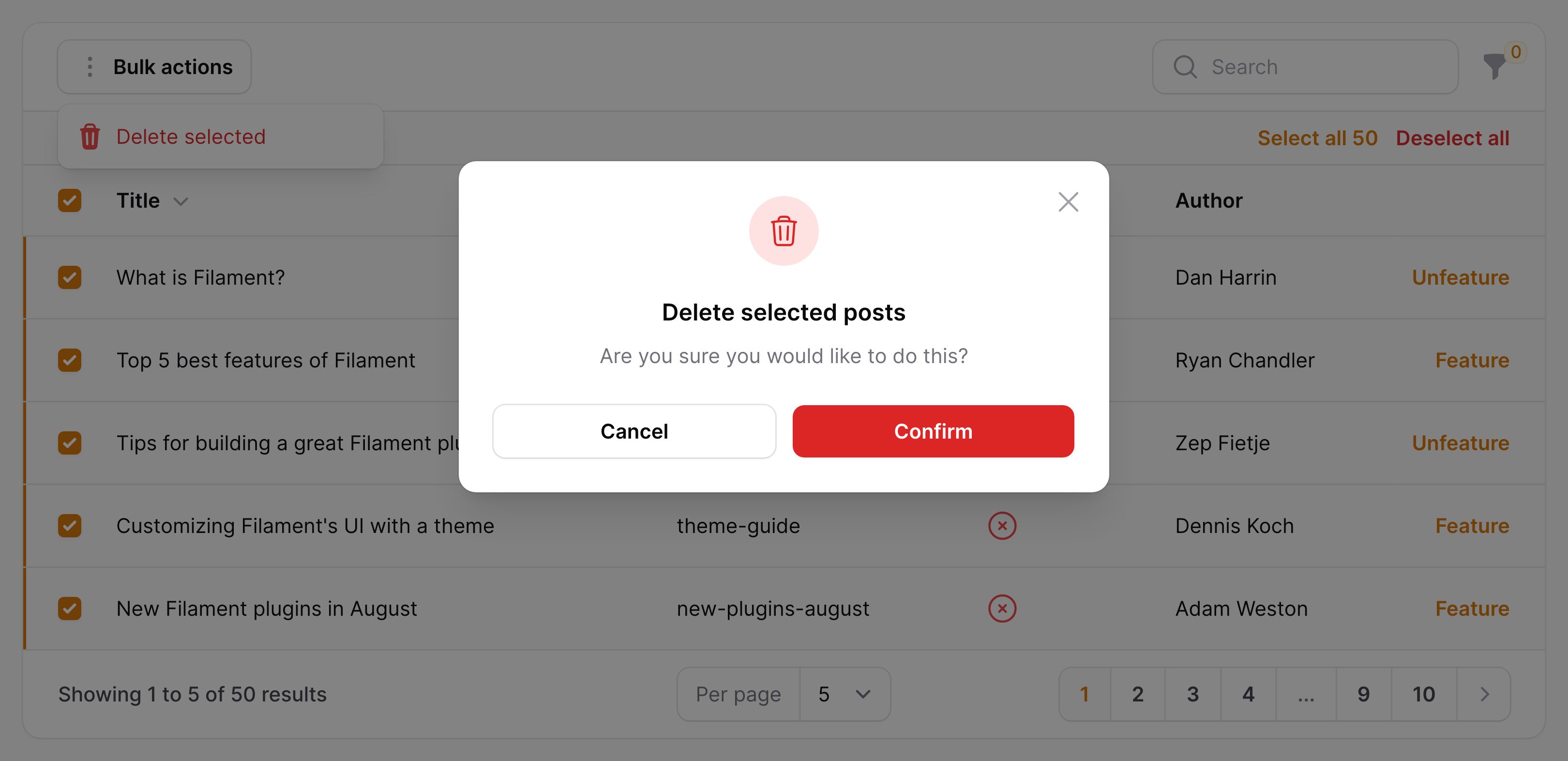
Actions can also open modals to request confirmation from the user, as well as render forms inside to collect extra data. It's a good idea to read the Actions documentation to learn more about their extensive capabilities throughout Filament.
Next steps with the Table Builder package
Now you've finished reading this guide, where to next? Here are some suggestions:
- Explore the available columns to display data in your table.
- Deep dive into table actions and start using modals.
- Discover how to build complex, responsive table layouts without touching CSS.
- Add summaries to your tables, which give an overview of the data inside them.
- Find out about all advanced techniques that you can customize tables to your needs.
- Write automated tests for your tables using our suite of helper methods.
Still need help? Join our Discord community or open a GitHub discussion